Python has become a popular programming language for game development due to its simplicity, versatility, and extensive libraries and frameworks. In this article, we will explore how Python can be used to create interactive experiences in game development.
Python Game Development Libraries
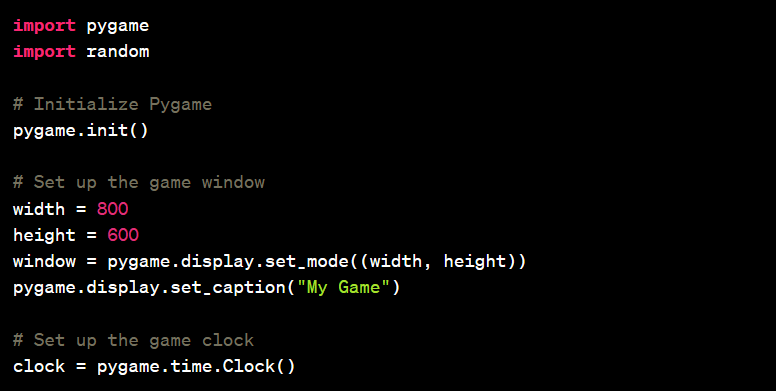
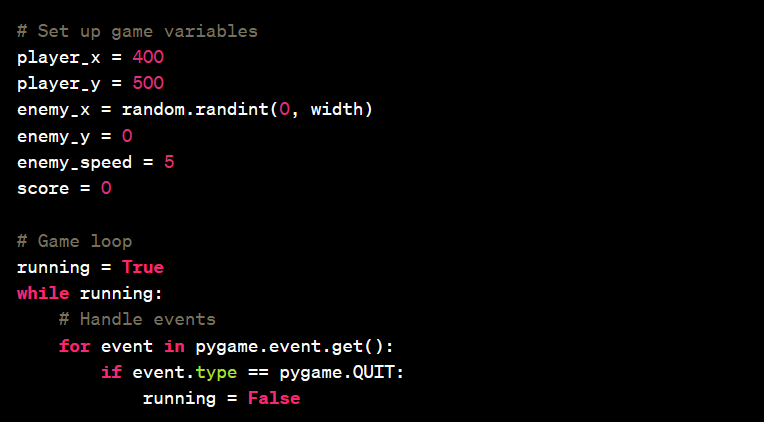
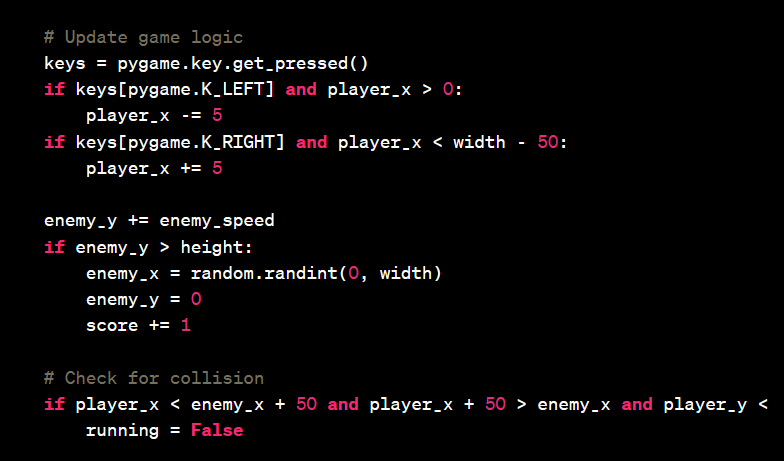
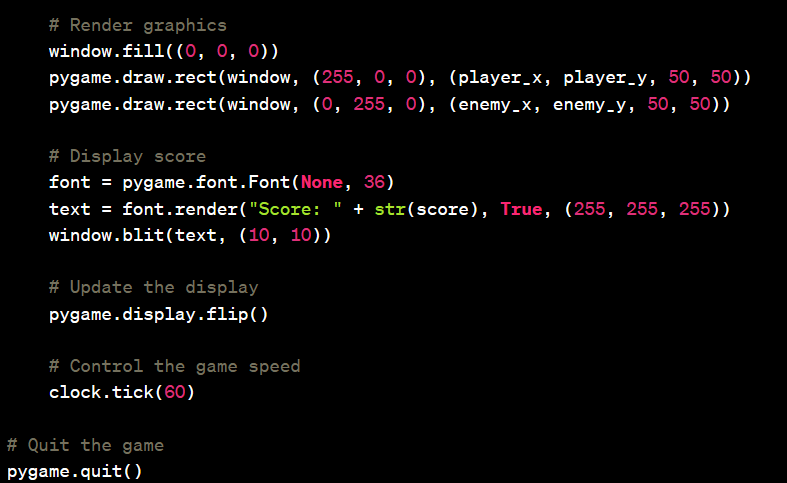
We utilize the Pygame library to create a basic game. The game window is set up with a defined width and height. The game loop continuously handles events, updates the game logic, and renders graphics.
The player is controlled using the left and right arrow keys, while an enemy object falls from the top of the screen. The game ends if the player collides with the enemy object. The score is displayed on the screen, and the game runs at 60 frames per second.
This is just a simple example, but Pygame provides a wide range of functionalities for building more complex games, including sprite handling, collision detection, sound effects, and more.
With Python and libraries like Pygame, you can unleash your creativity and build exciting games.
Python offers several powerful libraries and frameworks specifically designed for game development. Some of the notable ones include:
Pygame
Pygame is a popular library for creating 2D games in Python. It provides functions and classes for handling game graphics, sound, and input, making it easy to create interactive experiences.
Panda3D
Panda3D is a robust game engine that allows developers to create both 2D and 3D games. It provides high-level abstractions for rendering, physics, and networking, making it suitable for complex game projects.
Arcade
Arcade is a simple and beginner-friendly game development library for Python. It focuses on 2D games and provides a streamlined interface for creating game objects, handling input, and managing game states.
Game Development Concepts
To create engaging and interactive experiences in games, developers should understand key game development concepts. Some important concepts include:
Game Loop
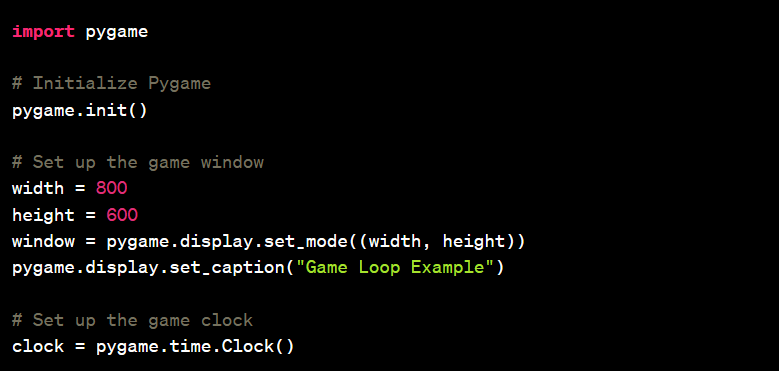
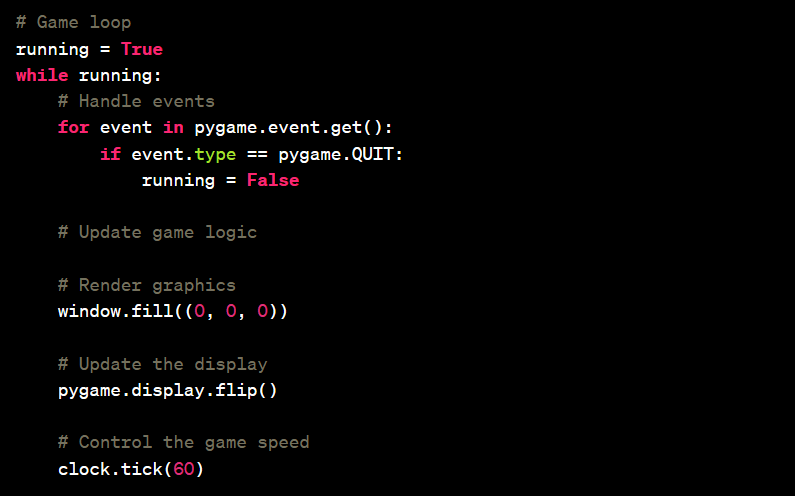
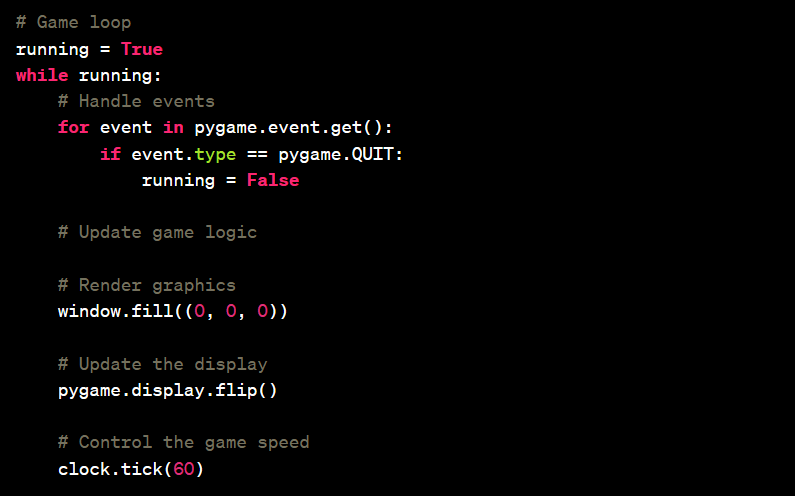
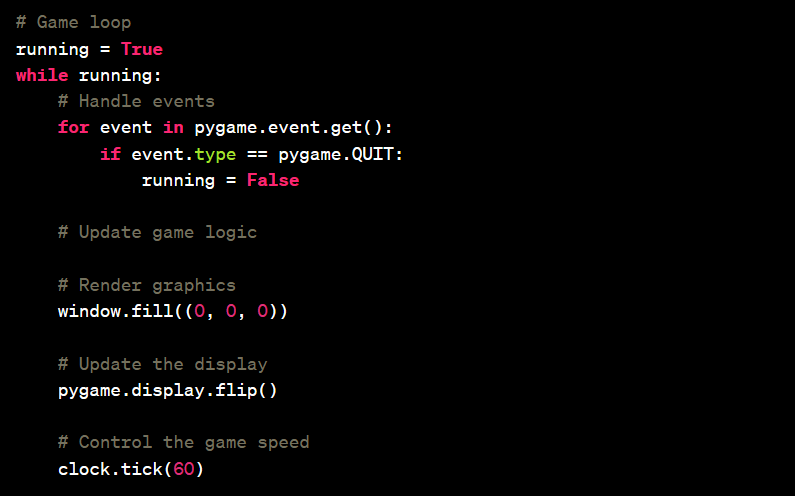
In this example, we have a basic game loop structure. The game loop runs continuously until the running variable becomes False. Inside the loop, we handle events using pygame.event.get() to check for the QUIT event, which is triggered when the user tries to close the game window.
If the QUIT event is detected, we set running to False to exit the loop and quit the game.
After handling events, we update the game logic. This is where you would implement any game mechanics, such as moving characters, detecting collisions, updating scores, etc.
In this example, the game logic section is left empty, but you can add your own custom game logic here.
Next, we render graphics on the game window using window.fill() to fill the window with a black color in this case. You can draw shapes, sprites, backgrounds, or any visual elements of your game within this section.
Finally, we update the display using pygame.display.flip() to reflect the changes made to the game window. The clock.tick(60) controls the frame rate of the game, ensuring it runs at approximately 60 frames per second.
This game loop structure allows the game to continuously handle events, update the game state, render graphics, and control the frame rate, creating a smooth and responsive gaming experience.
The game loop is a fundamental structure in game development that controls the flow of the game. It typically consists of three stages: processing user input, updating the game state, and rendering the game graphics.
Collision Detection:
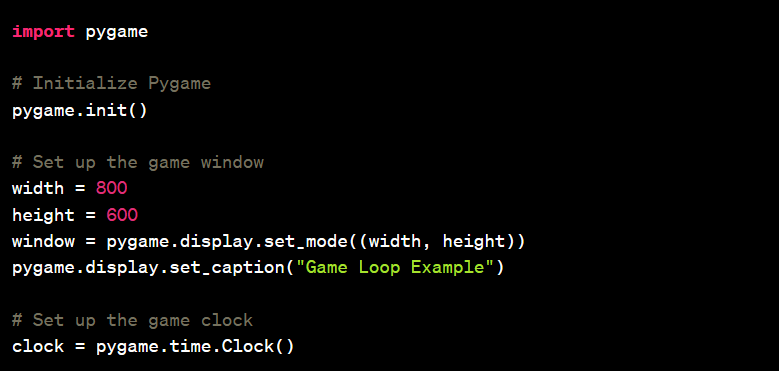
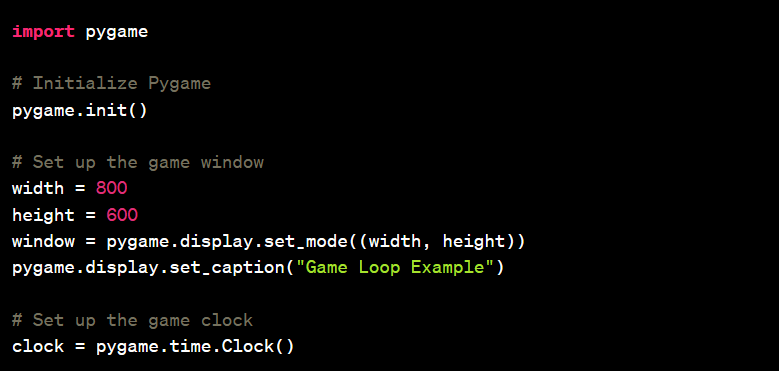
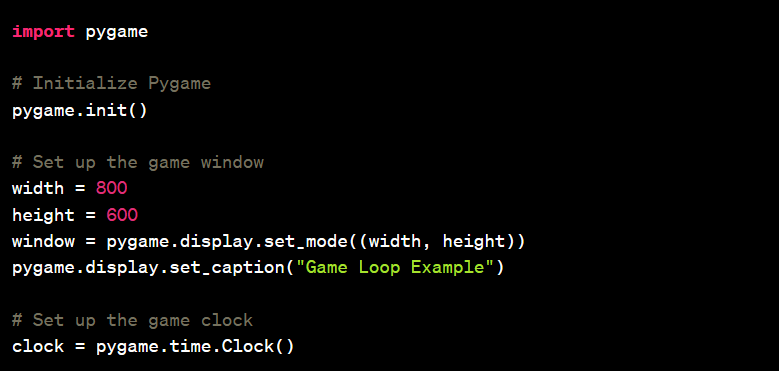
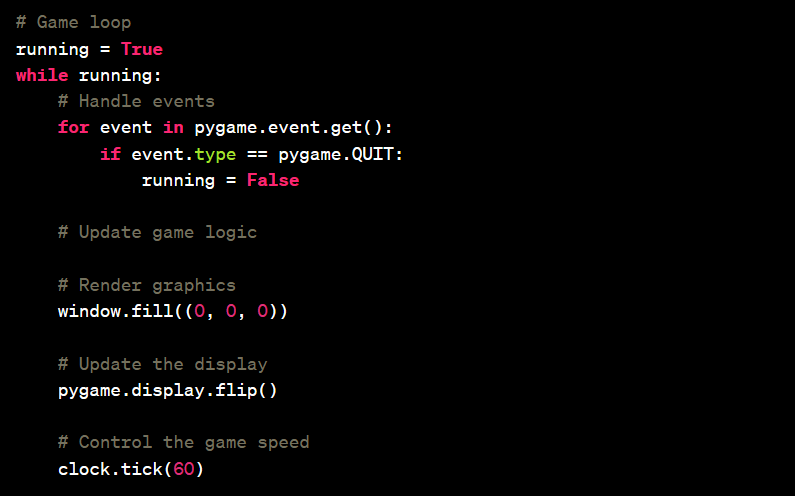
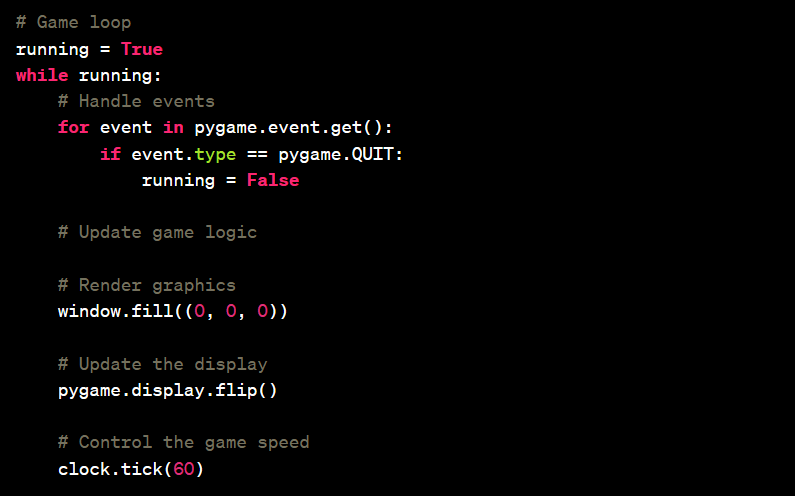
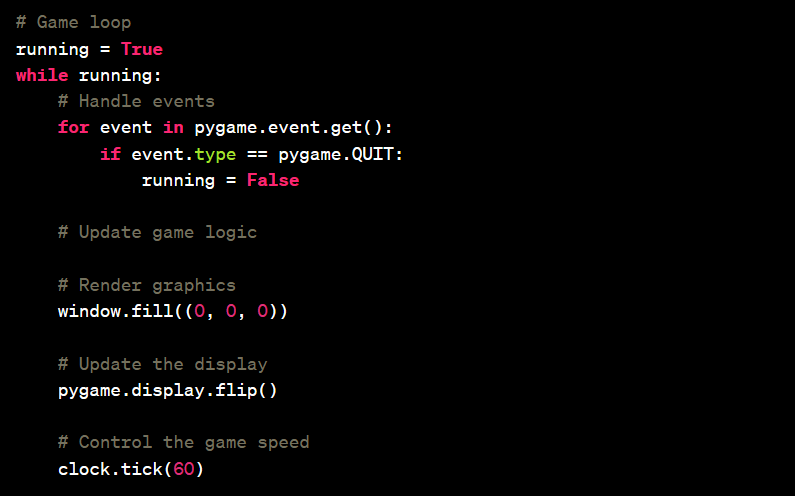
In this example, we have a basic game loop with collision detection. We define two rectangles, player_rect and enemy_rect, using the pygame.Rect class. These rectangles represent the player and enemy objects in our game.
Inside the game loop, after handling events, we check for collision using the colliderect() method of the pygame.Rect class. If the player_rect and enemy_rect collide, we print “Collision Detected!” to the console.
You can replace the print statement with your desired collision handling logic, such as decreasing health, adding points, or initiating game over conditions.
In the graphics rendering section, we fill the game window with a black color using window.fill() and draw the player and enemy rectangles using pygame.draw.rect().
The player rectangle is drawn in red, and the enemy rectangle is drawn in green for visual distinction.
Finally, we update the display using pygame.display.flip() to reflect the changes made to the game window.
This example demonstrates a simple collision detection mechanism using bounding rectangles. However, collision detection can be more complex depending on the requirements of your game.
Pygame provides additional features and techniques for more advanced collision detection, such as pixel perfect collision detection, circle collision, or using masks.
Collision detection is essential for games involving objects that interact with each other. Python libraries like Pygame provide built-in collision detection mechanisms, simplifying the implementation of interactive elements.
Animation and Graphics
Python libraries often offer features for rendering graphics and creating animations. These capabilities allow developers to bring game worlds to life and provide immersive experiences for players.
Python for Robotics, Building Intelligent Machines
Python is widely used in the field of robotics due to its ease of use and extensive libraries for hardware interfacing and control. Here, we will discuss how Python can be leveraged for building intelligent machines.
Robotics Frameworks
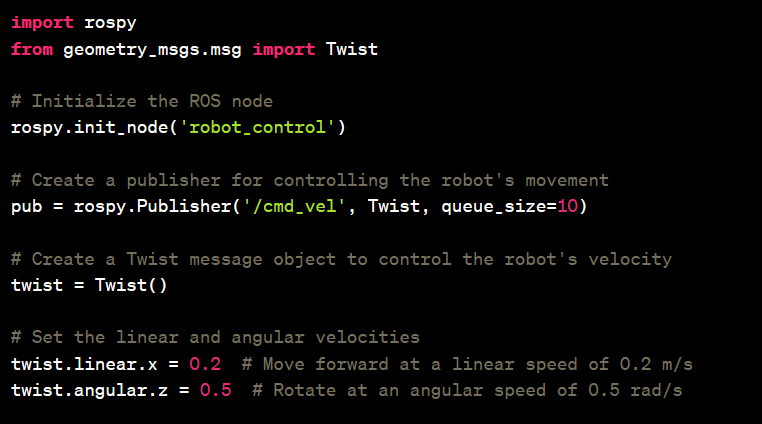
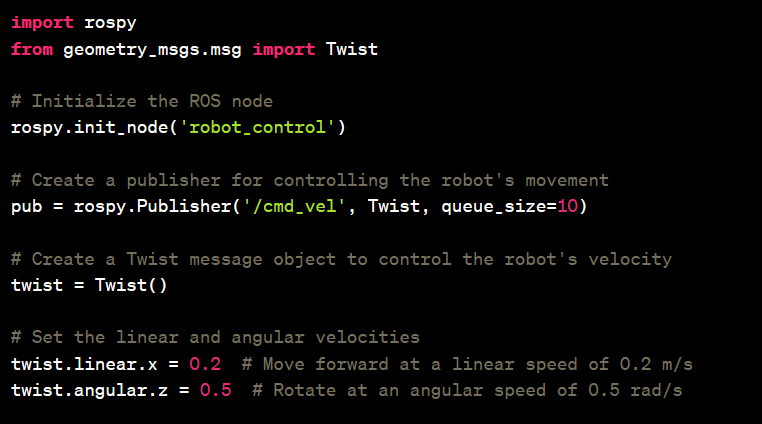
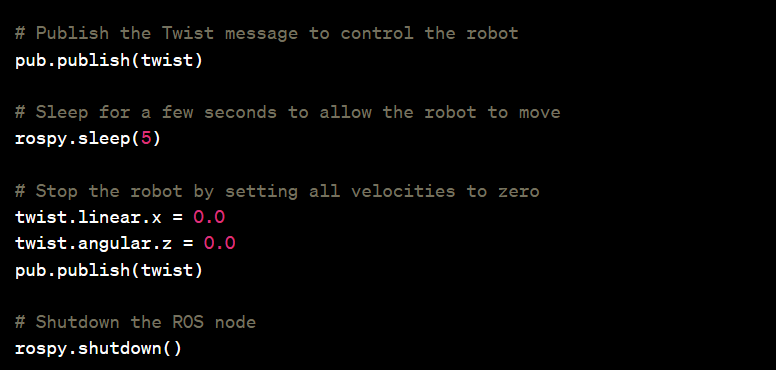
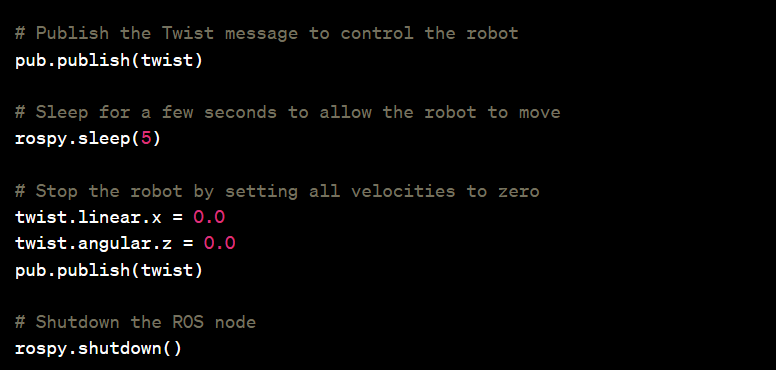
In this example, we use the ROS framework to control a robot’s movement. First, we initialize the ROS node using rospy.init_node(), giving it a unique name ‘robot_control’.
Next, we create a publisher pub that sends Twist messages on the ‘/cmd_vel’ topic. The Twist message is defined in the geometry_msgs.msg module and contains linear and angular velocity components.
We set the linear and angular velocities of the robot by modifying the twist object. In this example, we set a linear velocity of 0.2 m/s to move the robot forward and an angular velocity of 0.5 rad/s to rotate the robot.
We then publish the Twist message on the ‘/cmd_vel’ topic using pub.publish(twist). This controls the robot’s movement according to the specified velocities.
After a brief pause of 5 seconds using rospy.sleep(), we stop the robot by setting all velocities to zero.
Finally, we shut down the ROS node using rospy.shutdown().
This example demonstrates a simple robot control program using the ROS framework. ROS provides a comprehensive set of tools, libraries, and communication protocols to develop robotics applications, enabling developers to create complex robot behaviors and interact with various sensors and actuators.
Python-based frameworks like ROS (Robot Operating System) provide a flexible and modular approach to robotics development. ROS offers a wide range of libraries, tools, and algorithms for tasks such as perception, planning, and control.
Sensor Integration
Python libraries make it easier to interface with various sensors commonly used in robotics, such as cameras, lidars, and IMUs (Inertial Measurement Units). These libraries simplify data acquisition and processing.
Robot Control
Python enables developers to control robots and actuators by sending commands and receiving sensor feedback. This allows for the implementation of complex behaviors and intelligent decision-making algorithms.
Python for Network Programming and Cybersecurity
Python’s versatility extends to network programming and cybersecurity. It’s simplicity and rich ecosystem of libraries make it a preferred choice for developing networking applications and implementing security measures.
Network Programming
Python provides built-in libraries like sockets and asyncio for creating networked applications. These libraries enable developers to establish connections, send and receive data, and handle various network protocols.
Web Scraping
Python’s libraries, such as Beautiful Soup and Scrapy, facilitate web scraping, which involves extracting data from websites. Web scraping can be used for various purposes, such as gathering information or monitoring online content.
Cybersecurity
Python offers libraries like Scapy and Paramiko that assist in network security tasks. Scapy allows for the creation and manipulation of network packets, while Paramiko provides tools for secure communication via SSH (Secure Shell).
Python for Big Data Analytics and Processing
Python has gained popularity in the field of big data analytics and processing due to its rich ecosystem of data manipulation and analysis libraries. It offers various tools for handling large datasets efficiently.
Data Analysis Libraries
Python libraries such as NumPy, Pandas, and SciPy provide powerful tools for data analysis, manipulation, and statistical computing. These libraries offer functions for handling large arrays and performing complex computations.
Distributed Computing
Python frameworks like Apache Spark and Dask enable distributed computing for processing large datasets across clusters of machines. These frameworks allow developers to harness the power of parallel computing for data-intensive tasks.
Machine Learning and AI
Python’s extensive libraries for machine learning, such as scikit-learn and TensorFlow, make it a preferred choice for data scientists. These libraries provide algorithms and tools for tasks such as classification, regression, and clustering.
Python for Image Processing and Computer Vision
Python offers a range of libraries for image processing and computer vision tasks. These libraries allow developers to manipulate and analyze images, as well as build intelligent systems capable of understanding visual information.
OpenCV
OpenCV is a popular computer vision library that provides extensive tools for image and video analysis. It offers functions for image filtering, feature detection, object recognition, and more.
Pillow
The pillow is a user-friendly library for image-processing tasks. It provides functions for image manipulation, such as resizing, cropping, and applying various filters. The pillow is widely used in applications that involve image editing and enhancement.
Deep Learning and Convolutional Neural Networks (CNNs)
Python libraries like TensorFlow and PyTorch enable developers to build and train deep-learning models for image recognition and computer vision tasks. CNNs have shown remarkable success in tasks such as object detection and image segmentation.
Exploring Python’s Functional Programming Paradigm
Python supports multiple programming paradigms, including object-oriented programming (OOP) and functional programming (FP). Understanding functional programming in Python can lead to cleaner and more concise code.
Functional Programming Concepts
Functional programming focuses on writing programs by composing pure functions, avoiding mutable state, and emphasizing immutability and data transformations. Concepts like higher-order functions, lambda expressions, and function composition play a significant role in functional programming.
Functional Libraries
Python offers functional programming libraries like functools and itertools, which provide higher-order functions, lazy evaluation, and tools for functional-style programming. These libraries enable developers to write functional code and take advantage of functional programming techniques.
Benefits of Functional Programming
Functional programming can improve code readability, maintainability, and testability. It also promotes modularity and code reusability by reducing dependencies on mutable state and side effects.
Python for Web APIs and RESTful Services
Python’s simplicity and extensive libraries make it an excellent choice for building web APIs and RESTful services. These services enable communication between different systems over the internet.
Flask and Django
Flask and Django are popular Python frameworks for building web applications and APIs. Flask is lightweight and provides flexibility, while Django is a full-featured framework that includes ORM (Object-Relational Mapping) and authentication capabilities.
Python for Web APIs and RESTful Services
The proliferation of web-based applications and services has made Web APIs and RESTful services crucial components of modern software development. Python offers a robust ecosystem to build and consume APIs efficiently.
In 2023, popular Python libraries such as Flask, Django, and FastAPI have evolved to simplify API development. These frameworks provide seamless integration with databases, authentication mechanisms, and request handling, making it easier than ever to create scalable and secure APIs.
Additionally, libraries like requests, aiohttp, and httpx facilitate easy consumption of APIs, enabling developers to fetch and process data from external sources seamlessly.
Python for Financial Analysis and Algorithmic Trading
Python’s extensive libraries and tools have solidified its position in the financial industry, enabling sophisticated analysis and algorithmic trading strategies. In 2023, Python libraries such as Pandas, NumPy, and SciPy continue to be the backbone for data manipulation, statistical analysis, and numerical computations in financial research.
The advent of libraries like PyTorch and TensorFlow has empowered the use of machine learning models for predictive analytics, risk assessment, and algorithmic trading. Moreover, platforms like Quantopian and Zipline offer comprehensive environments for developing and backtesting trading strategies using Python.
Python for Natural Language Generation (NLG) and Text-to-Speech (TTS)
With advancements in natural language processing (NLP) and machine learning, Python has become a leading language for Natural Language Generation (NLG) and Text-to-Speech (TTS) applications.
In 2023, libraries such as NLTK, spaCy, and Hugging Face’s Transformers provide powerful tools for text preprocessing, semantic analysis, and language generation.
These libraries enable developers to create chatbots, automated content generation systems, and virtual assistants with ease. Moreover, Python libraries like pyttsx3 and gTTS offer seamless integration with text-to-speech engines, allowing developers to convert text into human-like speech.
Best Practices for Python Code Organization and Documentation
Maintaining clean, organized, and well-documented code is crucial for long-term project scalability and collaboration. In 2023, Python developers have embraced various best practices and tools to enhance code organization and documentation.
Popular code organization patterns like the Model-View-Controller (MVC) and Package-by-Feature have gained traction. Additionally, tools like Flake8, Black, and Pylint ensure code quality, enforce coding style guidelines, and identify potential errors and inefficiencies.
For documentation, Python developers widely utilize tools like Sphinx to generate professional-looking documentation from docstrings, enabling easy code comprehension and API reference for other developers.
Conclusion
Python’s dominance in the technology landscape of 2023 is undeniable, especially in the areas of Web APIs, Financial Analysis, NLG, and Code Organization.
The language’s vast library ecosystem, coupled with its simplicity and readability, makes Python an ideal choice for these cutting-edge applications. As developers continue to push the boundaries of Python’s capabilities,
it is essential to adopt best practices for code organization and documentation, ensuring maintainable and scalable projects. With Python’s ever-evolving technology landscape, the possibilities for innovation and development are boundless.