For Robust & Scalable Applications
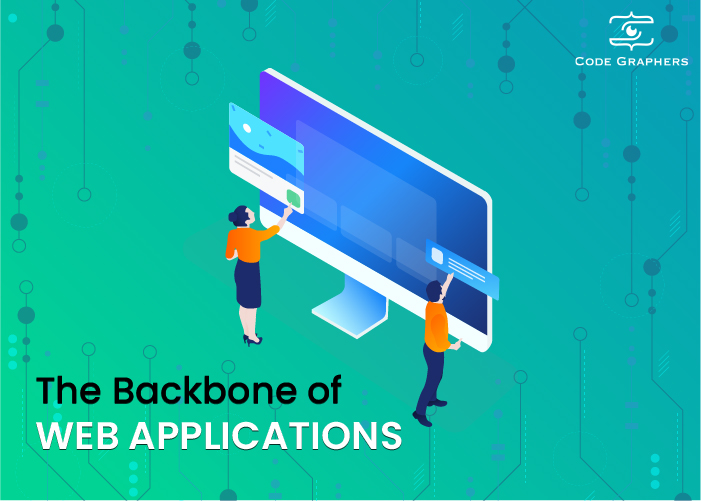
Spring is a powerful and widely used Java framework that provides comprehensive support for building robust and scalable enterprise applications. It offers a lightweight and modular approach to application development, emphasizing on the principles of dependency injection, aspect-oriented programming, and loose coupling.
In this essay, we will delve into the details of Spring, its purpose, and its key features, while providing examples to illustrate its usage.
At its core, Spring provides a container known as the “Spring IoC Container” (IoC stands for Inversion of Control). The IoC container is responsible for managing the lifecycle and configuration of application objects.
It achieves this by utilizing dependency injection, a design pattern that promotes loose coupling and facilitates the modular development of software components.
One of the primary objectives of Spring is to address the challenges associated with the traditional approach of writing enterprise applications, such as tight coupling between components, complex configuration, and difficulty in testing. By leveraging Spring’s features, developers can achieve greater flexibility, maintainability, and testability in their applications.
Let’s explore a few key features of Spring:
Dependency Injection: Spring facilitates dependency injection by automatically wiring the dependencies of an object, reducing the need for manual object creation and initialization. This helps in decoupling the components, making them easier to test and modify.
Here’s an example:
java Copy code public class CustomerService { private OrderService orderService; public CustomerService(OrderService orderService) { this.orderService = orderService; } // ... }
In this example, the CustomerService class has a dependency on the OrderService class. Spring will automatically inject an instance of OrderService when creating an instance of CustomerService, eliminating the need for manual instantiation.
Aspect-Oriented Programming (AOP): Spring integrates AOP, allowing developers to modularize cross-cutting concerns such as logging, security, and transaction management. AOP enables the separation of these concerns from the core business logic.
Here’s an example of configuring an aspect in Spring:
java Copy code @Aspect @Component public class LoggingAspect { @Before("execution(* com.example.service.*.*(..))") public void logMethodExecution(JoinPoint joinPoint) { String methodName = joinPoint.getSignature().getName(); System.out.println("Executing method: " + methodName); } }
In this example, the LoggingAspect class defines an advice that runs before any method execution in the com.example.service package. It prints a log message indicating the method being executed.
Spring MVC: Spring provides a robust web framework called Spring MVC, which simplifies the development of web applications. It follows the Model-View-Controller architectural pattern, allowing developers to build scalable and maintainable web applications.
Here’s an example of a Spring MVC controller:
java Copy code @Controller @RequestMapping("/products") public class ProductController { @Autowired private ProductService productService; @GetMapping public String getAllProducts(Model model) { List<Product> products = productService.getAllProducts(); model.addAttribute("products", products); return "product-list"; } // ... }
In this example, the ProductController class handles requests related to products. The @GetMapping annotation maps the method to handle HTTP GET requests for the “/products” endpoint.
It retrieves the products from the ProductService and adds them to the model before returning the view name “product-list”.
These are just a few highlights of the vast capabilities of the Spring framework. Spring also offers support for transaction management, database access through frameworks like Spring Data, integration with messaging systems, and much more.
In conclusion, Spring is a comprehensive Java framework that simplifies enterprise application development by providing features like dependency injection, aspect-oriented programming, and a powerful web framework.
It promotes modular and maintainable code, making it easier for developers to build robust and scalable applications. With its extensive set of features and strong community support, Spring has become one of the most popular frameworks for Java development.
Please note that the provided code examples are simplified for the sake of brevity and may not represent the complete setup or implementation of the respective features in a real-world scenario.